Understanding CWE-476: NULL Pointer Dereference
A Comprehensive Guide for Software Developers and Architects
The CWE-476 NULL Pointer Dereference is one of the most critical software weaknesses, consistently appearing in the 2024 CWE Top 25 Most Dangerous Software Weaknesses list. Its presence highlights the ongoing challenges developers face in ensuring robust, secure software systems. In this post, we will explore the intricacies of NULL Pointer Dereference, its implications, and practical strategies to mitigate the associated risks.
What is NULL Pointer Dereference?
In software development, a NULL pointer is a pointer variable that does not reference any valid memory location. Dereferencing such a pointer—attempting to access the memory it supposedly points to—results in undefined behaviour. In many systems, this leads to crashes, data corruption, or even exploitable vulnerabilities.
For example:
int *ptr = NULL;
printf(“%d”, *ptr); // Dereferencing a NULL pointer
This code will likely result in a runtime error or crash.
Why Does NULL Pointer Dereference Matter?
Business Impact
NULL pointer dereference can disrupt software functionality, leading to system crashes, downtime, and loss of customer trust. For industries reliant on software, such as finance or healthcare, these disruptions can have severe financial and reputational consequences.
Security Implications
While often perceived as a reliability issue, NULL pointer dereference can also create attack vectors for adversaries. In specific contexts, attackers exploit this weakness to execute code, escalate privileges, or cause denial-of-service (DoS).
Anatomy of the Issue: How NULL Pointer Dereferences Arise
Common Causes
Improper Initialisation:
Failing to initialise pointers appropriately can leave them pointing to arbitrary or NULL addresses.
int *ptr; // Uninitialised pointer
*ptr = 42; // Dereferencing uninitialised pointer
Unchecked Function Return Values:
Functions that return pointers might indicate errors using a NULL value. Developers often overlook checks for such conditions.
int *ptr = malloc(sizeof(int));
if (!ptr) {
printf(“Memory allocation failed\\n”);
}
Premature Deallocation:
Accessing memory after it has been freed can cause undefined behaviour.
free(ptr);
*ptr = 42; // Dereferencing after free
- Invalid Memory Access:
Array overflows or pointer arithmetic errors can inadvertently result in NULL references.
Real-World Examples of Impact
- Operating System Crashes:
Many OS kernel vulnerabilities arise from dereferencing NULL pointers. Such vulnerabilities can cause system-wide failures. - Web Application Downtime:
A NULL pointer dereference in server software can crash critical services, leading to revenue loss and customer dissatisfaction. - Industrial Systems Failures:
Embedded systems in vehicles or medical devices, when exposed to NULL pointer bugs, can malfunction, risking human safety.
Real-World Cyber Incidents Involving NULL Pointer Dereference (CWE-476)
NULL Pointer Dereference vulnerabilities have been at the centre of several high-profile cyber incidents. Below are notable examples that highlight the impact of CWE-476:
1. Microsoft Windows Kernel Vulnerability (CVE-2017-0263)
- Description: A NULL pointer dereference vulnerability in the Windows kernel was exploited to execute arbitrary code.
- Impact: This vulnerability was used in the FinFisher malware campaign, targeting high-profile individuals for surveillance.
- Business Impact: Sensitive data was exposed, compromising the privacy of users and damaging trust in the platform.
- Mitigation: Microsoft patched the vulnerability and introduced stricter validation checks in the kernel codebase.
2. OpenSSL NULL Pointer Dereference (CVE-2021-3449)
- Description: OpenSSL, a widely used cryptographic library, suffered from a NULL pointer dereference vulnerability in its TLS server. A maliciously crafted renegotiation request could cause the server to crash, leading to denial-of-service (DoS).
- Impact: This vulnerability affected millions of web servers globally, disrupting services and exposing businesses to downtime.
- Business Impact: The incident highlighted the need for continuous monitoring and patching in enterprise environments.
- Mitigation: Organisations updated to patched OpenSSL versions, mitigating the risk of future exploitation.
3. Android Bluetooth Vulnerability (CVE-2020-0022)
- Description: A NULL pointer dereference vulnerability in the Bluetooth stack of Android allowed attackers within range to execute code on the device.
- Impact: Devices running older versions of Android were vulnerable to unauthorised access and control.
- Business Impact: This affected millions of Android devices, including those used by enterprises, leading to potential data breaches and privacy violations.
- Mitigation: Google released a security update to fix the issue, urging users to upgrade their devices.
4. Apple macOS Kernel Vulnerability (CVE-2020-9893)
- Description: A NULL pointer dereference issue in macOS allowed attackers to crash the operating system or execute arbitrary code with kernel privileges.
- Impact: Exploited in targeted attacks, this vulnerability affected macOS devices in enterprise settings.
- Business Impact: The vulnerability highlighted gaps in macOS’s kernel security, leading to potential reputational damage for Apple among security-conscious customers.
- Mitigation: Apple issued security updates and revised its kernel coding practices.
5. PHP-FPM Vulnerability (CVE-2019-11043)
- Description: A NULL pointer dereference flaw in PHP-FPM (FastCGI Process Manager) allowed attackers to crash web applications or execute code remotely.
- Impact: Many web servers were targeted in real-world attacks, leading to service disruptions.
- Business Impact: Organisations relying on PHP-based applications faced downtime and potential data breaches.
- Mitigation: Developers were advised to update PHP-FPM configurations and apply the necessary patches.
6. Linux Kernel Issue (CVE-2021-26708)
- Description: A NULL pointer dereference in the Linux kernel’s virtual socket implementation allowed attackers to trigger a DoS attack or potentially escalate privileges.
- Impact: Public-facing servers running vulnerable Linux distributions were targeted, causing service disruptions.
- Business Impact: This highlighted the critical need for timely patching in large-scale enterprise environments using Linux.
- Mitigation: Updates to the Linux kernel were released, along with guidance for administrators to address the issue.
7. Cisco NX-OS Software Vulnerability (CVE-2018-0304)
- Description: A NULL pointer dereference vulnerability in Cisco’s NX-OS software could cause devices to reload, resulting in a denial-of-service condition.
- Impact: This issue was exploitable remotely, affecting critical networking devices in enterprise environments.
- Business Impact: Organisations relying on Cisco hardware faced significant downtime, affecting operations and customer services.
- Mitigation: Cisco released firmware updates to address the vulnerability and recommended best practices for device configuration.
8. VMware Workstation Pro NULL Pointer Dereference (CVE-2022-22938)
- Description: A NULL pointer dereference vulnerability in VMware Workstation Pro allowed attackers to crash the application or execute code.
- Impact: Exploited in environments where VMware was used for virtualisation, leading to system disruptions.
- Business Impact: Virtualised environments, often critical for enterprise operations, were left vulnerable to targeted attacks.
- Mitigation: VMware released an updated version of the software to fix the flaw.
Key Lessons from These Incidents
- Proactive Patch Management:
Regularly updating software is crucial to address NULL pointer dereference vulnerabilities promptly. - Code Quality Assurance:
Conducting rigorous code reviews and static analysis can help identify and mitigate such issues during development. - Security Awareness:
Training developers to understand and avoid common pitfalls like NULL pointer dereference can reduce vulnerabilities in production code. - Incident Response Readiness:
Preparing for swift action when vulnerabilities are disclosed can minimise the impact of real-world attacks.
By learning from these incidents, organisations can adopt best practices to prevent and mitigate NULL pointer dereference vulnerabilities effectively.
Prevention and Mitigation Strategies
Defensive Programming Practices
Initialise Pointers
Always initialise pointers to a valid memory address or explicitly set them to NULL if no memory is allocated.
int *ptr = NULL;
Validate Inputs and Outputs
Before dereferencing pointers, verify that they are not NULL. Use assertions where applicable.
if (ptr == NULL) {
printf(“Pointer is NULL\\n”);
return;
}
Adopt Safe Memory Management
Use smart pointers in languages like C++ to manage memory automatically. Smart pointers mitigate the risk of memory mismanagement.
std::unique_ptr<int> ptr = std::make_unique<int>(42);
- Code Reviews and Static Analysis
Incorporate code reviews and static analysis tools to identify potential NULL pointer dereference issues. Tools like Coverity, SonarQube, and Clang Static Analyzer are effective. - Unit Testing
Write comprehensive unit tests to simulate scenarios where pointers might be NULL, ensuring the code gracefully handles such cases.
Leveraging Modern Programming Languages
Certain modern programming languages, such as Rust and Swift, are designed to minimise the risk of NULL pointer dereference:
- Rust: Utilises an ownership model, where the compiler ensures memory safety.
- Swift: Employs optional types (Optional), explicitly requiring developers to handle cases where a variable might be nil.
NULL Pointer Dereference in Architecture
For software architects, preventing NULL pointer dereference involves designing systems with:
- Error Propagation Mechanisms:
Use structured error propagation, such as Result or Either types, to handle errors gracefully without resorting to NULL values. - Memory Safety Standards:
Define and enforce memory safety standards across development teams, incorporating best practices and tools. - Resilient Design Patterns:
Implement patterns like the Null Object Pattern, which provides default behaviours for objects to eliminate the use of NULL.
Advanced Techniques for Risk Mitigation
- Pointer Provenance Tracking:
Modern compilers can track the provenance of pointers to identify potential dereference risks during compilation. - Runtime Monitoring:
Use AddressSanitizer and similar tools to detect NULL pointer dereferences during runtime. - Dynamic Analysis:
Tools like Valgrind can dynamically analyse code for memory access errors, including NULL pointer dereferences.
Detecting NULL dereference vulnerabilities is critical in ensuring software security and reliability. A variety of tools, ranging from static analyzers to dynamic runtime monitoring utilities, are available for this purpose. Below is a list of tools and their features to help developers and architects identify NULL pointer dereference issues.
Static Analysis Tools
Static analysis tools examine source code without executing it, identifying potential NULL pointer dereference issues at compile-time.
1. Coverity
- Description: A static analysis tool that identifies NULL pointer dereferences, buffer overflows, and other common coding errors.
- Features:
- Deep semantic analysis.
- Automated triaging of results.
- Integration with CI/CD pipelines.
- Use Case: Large-scale projects requiring consistent and early detection of vulnerabilities.
2. SonarQube
- Description: A popular static code analysis platform with rulesets for detecting NULL pointer dereferences.
- Features:
- Supports multiple languages.
- Provides actionable insights with detailed code snippets.
- Integrates with development workflows.
- Use Case: Continuous code quality monitoring in agile environments.
3. Clang Static Analyzer
- Description: An open-source tool included with the Clang/LLVM compiler that identifies NULL pointer dereference and other defects.
- Features:
- Lightweight and fast.
- Provides detailed diagnostics for each issue.
- Supports C, C++, and Objective-C.
- Use Case: Projects using Clang for compilation.
4. CodeSonar
- Description: Advanced static analysis tool designed for safety-critical software.
- Features:
- Identifies NULL dereferences, race conditions, and memory leaks.
- Visualisation of data and control flows.
- Supports compliance with safety standards like MISRA.
- Use Case: High-assurance software in automotive or aerospace industries.
5. PVS-Studio
- Description: A static code analysis tool for C, C++, C#, and Java that detects NULL pointer dereferences.
- Features:
- User-friendly GUI.
- Customisable rule sets.
- Integration with popular IDEs.
- Use Case: Desktop application and embedded system development.
Dynamic Analysis Tools
Dynamic analysis tools detect NULL pointer dereferences by executing the code in a controlled environment.
6. Valgrind (Memcheck)
- Description: A dynamic analysis tool that detects memory management issues, including NULL dereferences.
- Features:
- Identifies invalid memory access in real-time.
- Produces detailed stack traces.
- Supports various Linux-based platforms.
- Use Case: Runtime testing of applications in development or production environments.
7. AddressSanitizer (ASan)
- Description: A fast memory error detector included with GCC and Clang.
- Features:
- Detects NULL dereferences, buffer overflows, and use-after-free errors.
- Lightweight and suitable for large projects.
- Minimal runtime overhead.
- Use Case: Testing software builds during the development phase.
8. Dr. Memory
- Description: A memory monitoring tool for identifying runtime errors such as NULL pointer dereferences.
- Features:
- Supports Windows, Linux, and macOS.
- Detects uninitialised memory accesses and memory leaks.
- Produces comprehensive error reports.
- Use Case: Debugging applications in diverse operating environments.
Integrated Development Environment (IDE) Features
Many modern IDEs offer built-in tools to detect potential NULL dereference vulnerabilities.
9. Visual Studio (Code Analysis)
- Description: Microsoft Visual Studio includes static code analysis features for detecting coding errors like NULL pointer dereferences.
- Features:
- Integrated into the IDE.
- Provides suggestions for fixes.
- Real-time feedback while coding.
- Use Case: Windows-based development projects.
10. Eclipse with FindBugs/SpotBugs
- Description: Eclipse IDE can integrate with SpotBugs, a static analysis tool for Java.
- Features:
- Detects NULL dereference issues in Java projects.
- Easy integration with build systems like Maven.
- Use Case: Java enterprise application development.
Hybrid Tools
Hybrid tools combine static and dynamic analysis techniques to detect vulnerabilities.
11. Klocwork
- Description: A hybrid analysis tool for C, C++, Java, and C# that detects NULL pointer dereferences and other issues.
- Features:
- Scales to large codebases.
- Real-time feedback for developers.
- Integrates with DevOps workflows.
- Use Case: Enterprises requiring end-to-end software quality solutions.
12. Infer
- Description: A static and dynamic analysis tool developed by Facebook.
- Features:
- Analyses code changes incrementally.
- Detects potential crashes, including NULL pointer dereferences.
- Open source and lightweight.
- Use Case: Agile teams managing frequent code updates.
Automated Security Testing Tools
13. Fortify Static Code Analyzer (SCA)
- Description: A comprehensive static analysis tool from Micro Focus for identifying security vulnerabilities, including NULL pointer dereferences.
- Features:
- Extensive security rulesets.
- Integrates with security testing frameworks.
- Detailed reporting and risk assessment.
- Use Case: Security-critical projects with high compliance requirements.
Selecting the Right Tool
- Project Size: Larger projects benefit from scalable tools like Coverity or Klocwork.
- Programming Language: Choose tools that support your primary language(s) (e.g., SonarQube for Java, PVS-Studio for C++).
- Budget: Open-source tools like Clang Static Analyzer and Infer are suitable for budget-conscious projects.
- Integration Needs: Tools like SonarQube and Coverity integrate seamlessly with CI/CD pipelines for continuous monitoring.
Investing in these tools can significantly enhance software reliability and security by identifying NULL pointer dereference vulnerabilities early in the development lifecycle.
Case Study: Preventing NULL Pointer Dereference in a Banking Application
In one notable instance, a banking application suffered a critical downtime due to a NULL pointer dereference in its transaction processing module. Here’s how the issue was resolved:
- Root Cause Analysis:
The NULL pointer originated from a failed database connection that was not adequately checked before use. - Mitigation:
Developers implemented strict pointer validation checks and replaced NULL checks with exceptions for better error handling. - Outcome:
Post-fix, the application achieved 99.99% uptime and reduced critical crashes by 90%.
The Role of Leadership in Addressing CWE-476
C-level executives play a vital role in mitigating the risks associated with NULL pointer dereference:
- Invest in Training:
Sponsor workshops and training sessions for development teams on secure coding practices. - Adopt Secure Development Frameworks:
Ensure teams use frameworks and libraries that prioritise memory safety. - Emphasise ROI:
Highlight the cost-benefit of proactive risk mitigation, such as reduced downtime and improved customer satisfaction.
Here is the comparative table in a tabular format:
Programming Language | NULL Pointer Handling | Strengths | Weaknesses |
C | Relies on manual checks; prone to errors and undefined behaviour. | High performance and low-level access to memory. | Highly prone to NULL pointer dereference if not handled manually. |
C++ | Offers smart pointers (e.g., std::unique_ptr, std::shared_ptr) to manage memory safely. | Combines performance with improved safety through smart pointers. | Smart pointers require additional knowledge to use effectively. |
Java | Uses null as a reference; throws NullPointerException if dereferenced. | Built-in exception handling for null references. | NullPointerException can still occur if not explicitly handled. |
Python | No pointers; uses None type with explicit handling. | Dynamic typing reduces pointer risks. | Potential runtime errors if None is not handled properly. |
Rust | Avoids NULL pointers entirely; uses Option type for optional values. | Compile-time memory safety; prevents NULL pointer dereferences. | Steeper learning curve for beginners. |
Swift | Uses Optional type to explicitly manage nil values. | Encourages safe handling of nil values. | Requires careful use of Optional to avoid nil errors. |
Go | Provides nil values; explicitly checks for safety. | Static typing and simplicity enhance safety. | Less suitable for low-level system programming. |
This table provides a clear and concise comparison of how different programming languages handle NULL pointers and their strengths and weaknesses.
Final Thoughts
The CWE-476 NULL Pointer Dereference remains a significant challenge for software developers and architects, but with the right tools, practices, and frameworks, it can be effectively mitigated. By embracing defensive programming, leveraging modern language features, and fostering a culture of secure coding, organisations can reduce the risk and impact of this vulnerability, ensuring reliable and secure software systems.
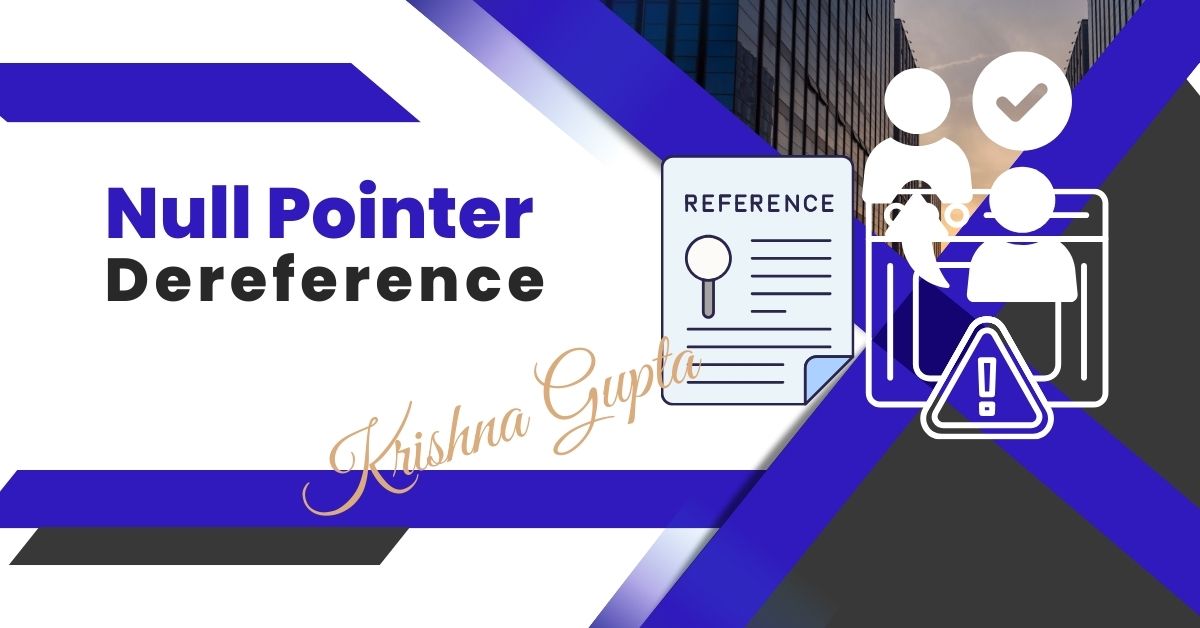
Addressing this weakness not only secures applications but also strengthens trust with customers and stakeholders, providing a measurable return on investment. Let this be a call to action for developers, architects, and executives to prioritise memory safety in their software development lifecycle.