The OWASP Top 10: Injection Vulnerabilities
Injection vulnerabilities rank among the most critical and persistent issues in web application security. Identified as one of the OWASP Top 10 security risks, these vulnerabilities pose significant threats to organisations of all sizes, potentially leading to data breaches, financial losses, and reputational damage. This blog post explores the nature of injection vulnerabilities, their impact, real-world examples, and mitigation strategies, all with a focus on empowering software developers to build more secure applications.
What Are Injection Vulnerabilities?
Injection vulnerabilities occur when untrusted data is incorporated into a query, command, or interpreter without proper validation or sanitisation. This flaw allows attackers to manipulate the application’s behaviour by injecting malicious inputs, which are processed as part of legitimate commands.
Common Types of Injection Vulnerabilities
- SQL Injection (SQLi):
- Attackers modify Structured Query Language (SQL) queries to manipulate databases.
- Example: Accessing unauthorised data by injecting OR ‘1’=’1′ into a login form.
- Command Injection:
- Malicious commands are executed on the server operating system.
- Example: Including ; rm -rf / in a parameter processed by a shell command.
- Cross-Site Scripting (XSS):
- Injecting malicious scripts into web pages viewed by users.
- Example: <script>alert(‘XSS’);</script> embedded into input fields.
- LDAP Injection:
- Manipulating Lightweight Directory Access Protocol (LDAP) queries.
- Example: Injecting *“ to bypass authentication checks.
- XML External Entity (XXE) Injection:
- Exploiting XML parsers to read local files or execute remote requests.
The Impact of Injection Vulnerabilities
1. Data Breaches and Theft
Injection flaws like SQLi allow attackers to exfiltrate sensitive information, including personal data, financial records, and trade secrets.
Example:
The 2014 cyberattack on eBay, which compromised 145 million accounts, involved injection vulnerabilities.
2. System Compromise
Command injection can enable attackers to execute arbitrary commands on the server, potentially leading to full system control.
Example:
In a 2020 incident, a vulnerability in a popular IoT device allowed attackers to install ransomware via command injection.
3. Reputational Damage
Exploited vulnerabilities often make headlines, eroding customer trust and confidence. Organisations face regulatory fines and legal challenges.
4. Financial Losses
For businesses, the costs of mitigating a breach, compensating affected customers, and dealing with legal consequences can be astronomical.
Why Injection Vulnerabilities Persist
- Lack of Input Validation: Developers often fail to validate or sanitise inputs adequately, trusting user-provided data.
- Complexity of Legacy Code: Older systems may lack modern security practices, making them vulnerable.
- Evolving Attack Techniques: Attackers continuously discover novel methods to exploit injection flaws.
- Inadequate Testing: Limited use of security-focused testing tools leaves vulnerabilities undiscovered until they are exploited.
Mitigation Strategies
To defend against injection attacks, developers must adopt a proactive, multi-layered security approach.
1. Parameterised Queries
- Use parameterised queries (e.g., prepared statements) to separate code from data.
- Avoid dynamically constructing SQL queries.
Example in Python:
python
cursor.execute(“SELECT * FROM users WHERE username = %s”, (username,))
2. Use ORM Frameworks
- Object-Relational Mapping (ORM) frameworks abstract database interactions, reducing direct query construction.
Example:
Frameworks like Hibernate or Django ORM inherently mitigate SQLi risks.
3. Input Validation and Sanitisation
- Ensure that inputs conform to expected formats and lengths.
- Escape special characters in input data.
4. Implement Secure Coding Practices
- Adhere to frameworks and libraries with built-in security features.
- Conduct code reviews to identify insecure patterns.
5. Content Security Policy (CSP)
- For XSS, enforce CSP headers to restrict the execution of unauthorised scripts.
Example CSP Header:
http
Content-Security-Policy: script-src ‘self’; object-src ‘none’;
6. Regular Testing
- Use automated tools like OWASP ZAP, Burp Suite, or custom scripts for testing injection vulnerabilities.
- Conduct penetration testing to uncover complex issues.
7. Logging and Monitoring
- Implement logging mechanisms to detect unusual patterns, such as multiple failed login attempts with injection payloads.
Real-World Case Studies
Case Study 1: SQL Injection in an E-commerce Application
A small online retailer’s login form was vulnerable to SQLi due to dynamic query construction. The attacker bypassed authentication, exfiltrating 10,000 customer records.
Resolution:
The company switched to prepared statements and implemented input validation to prevent similar attacks.
Case Study 2: Command Injection in a Cloud Service
A misconfigured endpoint accepted unsanitised user inputs, allowing an attacker to execute a shell command that deleted critical application files.
Resolution:
The organisation integrated strict input validation and sandboxing to isolate sensitive operations.
Practical Tips for Developers
- Adopt the Principle of Least Privilege: Restrict database user permissions to limit the damage caused by successful injection attacks.
- Automate Security Testing: Integrate tools like Snyk, Checkmarx, or Veracode into your CI/CD pipelines.
- Educate and Train: Regularly update developers on evolving security threats and best practices.
- Follow OWASP Guidelines: Leverage resources like the OWASP Cheat Sheet Series for comprehensive guidance.
Real-World Incidents of Injection Vulnerabilities
Injection vulnerabilities have been at the centre of some of the most notorious cyberattacks in history. These incidents underscore the devastating consequences of neglecting proper security measures. Below are some notable examples:
1. Equifax Data Breach (2017)
Attack Vector: SQL Injection
Impact: Compromise of sensitive data belonging to 147 million individuals, including Social Security numbers, birth dates, and addresses.
Details:
Attackers exploited a SQL injection vulnerability in a web application associated with Equifax’s consumer dispute portal. The flaw allowed unauthorised access to backend databases, enabling attackers to exfiltrate sensitive data over several months.
Lessons Learned:
- Timely patching of vulnerabilities is critical.
- A robust Web Application Firewall (WAF) could have mitigated the attack.
- Regular security audits and penetration testing could have identified the issue.
2. British Airways Data Breach (2018)
Attack Vector: Cross-Site Scripting (XSS)
Impact: Theft of personal and financial details of 400,000 customers.
Details:
Hackers exploited a vulnerability in the airline’s website to inject malicious scripts. The scripts intercepted payment card details entered by customers, redirecting them to a malicious server.
Lessons Learned:
- Implementing a strict Content Security Policy (CSP) could have limited the impact of XSS.
- Enhanced input sanitisation and validation could have prevented malicious script execution.
- Continuous monitoring and real-time threat detection are essential.
3. Sony Pictures Hack (2014)
Attack Vector: Command Injection
Impact: Leak of confidential data, including unreleased movies, employee information, and sensitive internal emails.
Details:
The attack involved exploiting command injection vulnerabilities in Sony’s IT infrastructure, allowing attackers to execute arbitrary commands and gain extensive control over systems. The breach was allegedly carried out by a nation-state actor.
Lessons Learned:
- Isolation of critical systems can limit the impact of such attacks.
- Implementing stricter input validation and sandboxing techniques can thwart command injection attempts.
- Regular threat modelling and red teaming exercises help identify vulnerabilities.
4. Heartland Payment Systems Breach (2008)
Attack Vector: SQL Injection
Impact: Theft of data from 134 million credit cards, resulting in losses exceeding $140 million.
Details:
Attackers exploited a SQL injection vulnerability in Heartland’s payment processing systems to install malware that intercepted payment data in transit.
Lessons Learned:
- Encrypting sensitive data at all stages, including transmission and storage, is crucial.
- Comprehensive logging and monitoring can help detect anomalies.
- Adopting secure development practices like input validation and parameterised queries can prevent SQLi.
5. Magento Vulnerability Exploited by Magecart (2015–2020)
Attack Vector: SQL Injection and Cross-Site Scripting
Impact: Hundreds of e-commerce sites compromised, leading to theft of payment card data.
Details:
Magecart, a hacking group, targeted vulnerabilities in Magento, a popular e-commerce platform. SQL injection and XSS were used to inject malicious scripts into payment pages, skimming cardholder data during transactions.
Lessons Learned:
- Applying patches promptly and keeping software updated is essential.
- Enforcing security headers and CSP reduces the impact of XSS attacks.
- Implementing a layered defence strategy, including endpoint protection, mitigates the risk of exploitation.
6. Drupalgeddon (2014, 2018)
Attack Vector: SQL Injection and Remote Command Execution
Impact: Compromise of hundreds of thousands of websites.
Details:
In 2014 and 2018, critical vulnerabilities in Drupal, a widely used content management system, allowed attackers to execute SQL injection and remote commands. These exploits provided access to sensitive data and enabled attackers to hijack affected websites.
Lessons Learned:
- Open-source platforms must be regularly monitored and patched.
- Organisations should monitor for vulnerabilities in third-party software.
- Automated security scans can detect and resolve issues before exploitation.
7. TalkTalk Breach (2015)
Attack Vector: SQL Injection
Impact: Exposure of personal data for over 156,000 customers, including bank account details.
Details:
The attacker exploited a SQL injection vulnerability on TalkTalk’s website, gaining unauthorised access to customer data. The breach highlighted poor cybersecurity practices, including inadequate data encryption and lack of regular security testing.
Lessons Learned:
- Strong encryption practices should be standard for sensitive data.
- Security testing should be a regular part of the development cycle.
- Public-facing applications must be subjected to regular penetration tests.
8. MySpace Data Breach (2006)
Attack Vector: Cross-Site Scripting (XSS)
Impact: Massive propagation of a worm that added millions of friends to an attacker’s account.
Details:
The Samy worm exploited an XSS vulnerability on MySpace profiles. It spread automatically, executing malicious JavaScript that added the creator to other users’ friend lists.
Lessons Learned:
- Output encoding and CSP implementation can mitigate XSS vulnerabilities.
- Social media platforms must adopt stringent input validation measures.
- Regular updates to security policies and frameworks are essential.
Common Themes Across Incidents
- Neglect of Input Validation: Almost all cases exploited poorly validated inputs.
- Delay in Applying Patches: Many attacks targeted known vulnerabilities in unpatched systems.
- Lack of Defence-in-Depth: Missing layered security mechanisms amplified the impact.
- Inadequate Monitoring: The inability to detect attacks early led to extensive damage.
Business Implications of Injection Vulnerabilities
From a business perspective, injection vulnerabilities jeopardise operational continuity, data integrity, and customer trust. Implementing robust security measures is not merely a technical necessity but a strategic imperative.
Return on Investment (ROI):
Proactively addressing injection risks through automated tools, secure coding practices, and regular audits significantly outweighs the costs of remediating breaches.
Risk Mitigation:
A secure application minimises the likelihood of regulatory fines and protects an organisation’s reputation in an increasingly digital economy.
Injection vulnerabilities remain a formidable challenge, but they are preventable with diligent effort. By adopting parameterised queries, using ORM frameworks, and incorporating robust security practices, developers can shield applications from exploitation. Regular testing and adherence to OWASP guidelines further strengthen an organisation’s security posture, safeguarding data and maintaining trust.
Addressing injection vulnerabilities is not just about technical measures; it’s a commitment to building a secure digital environment that benefits users, developers, and businesses alike.
Real-world incidents of injection vulnerabilities provide critical lessons for software developers. By understanding the patterns and impact of such attacks, organisations can adopt proactive measures to safeguard their systems. Regular updates, robust input validation, parameterised queries, and vigilant monitoring are indispensable in building resilient applications.
In today’s threat landscape, learning from past incidents is a powerful strategy to anticipate and mitigate future risks.
Preventing Injection Vulnerabilities:
Secure Code Reviews, Secure Software Development Life Cycle (SDLC), and Web Application Penetration Testing
Injection vulnerabilities remain among the most critical threats to web applications. To effectively mitigate these risks, organisations and developers must incorporate robust practices throughout the software development lifecycle. Here’s how secure code reviews, an SDLC approach, and penetration testing play pivotal roles in preventing injection vulnerabilities.
Secure Code Reviews
Definition:
Secure code reviews involve systematically examining source code to identify vulnerabilities, including injection flaws, before deployment. This process ensures that potential risks are addressed early, reducing the likelihood of exploitation in production.
How Code Reviews Help Prevent Injection Vulnerabilities
- Identification of Unsafe Code Patterns:
- Detects unvalidated user inputs, improper use of dynamic queries, and lack of parameterisation.
- Highlights instances of hard-coded credentials, which can exacerbate injection risks.
- Validation of Security Best Practices:
- Ensures adherence to secure coding standards, such as OWASP recommendations.
- Confirms that APIs and libraries are used securely.
- Collaboration and Knowledge Sharing:
- Encourages team discussions on secure coding practices.
- Provides opportunities for less experienced developers to learn secure techniques.
Best Practices for Secure Code Reviews
- Use static analysis tools like SonarQube or Checkmarx to automate detection of injection vulnerabilities.
- Employ checklists tailored to prevent injection flaws (e.g., ensuring parameterised queries and input sanitisation).
- Conduct reviews in tandem with testing phases to ensure comprehensive coverage.
- Focus on high-risk areas, such as database interactions and user input handling.
Secure Software Development Life Cycle (SDLC)
Definition:
An SDLC integrates security into every phase of software development, from planning to deployment, ensuring that applications are secure by design.
Phases of SDLC to Address Injection Vulnerabilities
- Planning:
- Incorporate security requirements in project specifications.
- Define standards for input validation, encoding, and output sanitisation.
- Design:
- Employ secure architectural patterns, such as least privilege and defence-in-depth.
- Use Object-Relational Mapping (ORM) frameworks to abstract database operations and prevent direct query manipulation.
- Development:
- Implement parameterised queries to prevent SQL Injection.
- Utilise secure coding frameworks like ESAPI or OWASP Cheat Sheet Guidelines.
- Use tools like Input Sanitisation Libraries to validate and encode user inputs.
- Testing:
- Perform dynamic and static analysis to identify vulnerabilities.
- Integrate automated security testing tools into the CI/CD pipeline, such as SAST and DAST.
- Deployment:
- Ensure that application servers are securely configured (e.g., disable unnecessary features).
- Deploy Web Application Firewalls (WAFs) to monitor and block malicious injection attempts.
- Maintenance:
- Regularly update dependencies and patch known vulnerabilities.
- Continuously monitor applications for new threats using tools like SIEM systems.
Web Application Penetration Testing
Definition:
Web application penetration testing (pentesting) simulates real-world attack scenarios to identify and mitigate vulnerabilities, including injection attacks.
Role of Penetration Testing in Preventing Injection Vulnerabilities
- Identification of Injection Points:
- Tests for SQL Injection, Command Injection, and XSS vulnerabilities by simulating malicious inputs.
- Ensures that APIs and microservices are resilient to injection attacks.
- Validation of Security Controls:
- Confirms that parameterised queries and stored procedures are implemented correctly.
- Verifies the effectiveness of input validation and sanitisation.
- Comprehensive Coverage:
- Assesses both client-side and server-side injection vulnerabilities.
- Evaluates third-party integrations and external libraries for weaknesses.
- Real-World Scenarios:
- Simulates advanced attack methods used by threat actors, such as union-based SQLi and blind XSS.
- Tests the application under different conditions, such as varying input lengths and encodings.
Best Practices for Web Application Pentesting
- Use tools like Burp Suite, OWASP ZAP, and SQLmap for automated and manual testing.
- Conduct tests on staging environments to avoid disrupting production systems.
- Perform regular pentests to keep pace with evolving threats.
- Involve certified testers with expertise in application security and injection vulnerabilities.
Integrating Practices for Maximum Effectiveness
- Collaboration Between Teams:
Secure code reviews, SDLC, and penetration testing should not operate in silos. Development, security, and testing teams must work together to ensure a seamless integration of these practices. - Continuous Improvement:
- Incorporate feedback from pentesting and code reviews into SDLC processes.
- Regularly update checklists and guidelines based on emerging injection threats.
- Education and Training:
- Provide developers with training on secure coding practices and common injection attacks.
- Conduct workshops to demonstrate real-world injection exploit scenarios and their mitigation.
- Automation:
- Use CI/CD pipelines to automate static code analysis, dynamic testing, and deployment checks.
- Integrate tools like GitHub Dependabot to identify insecure dependencies.
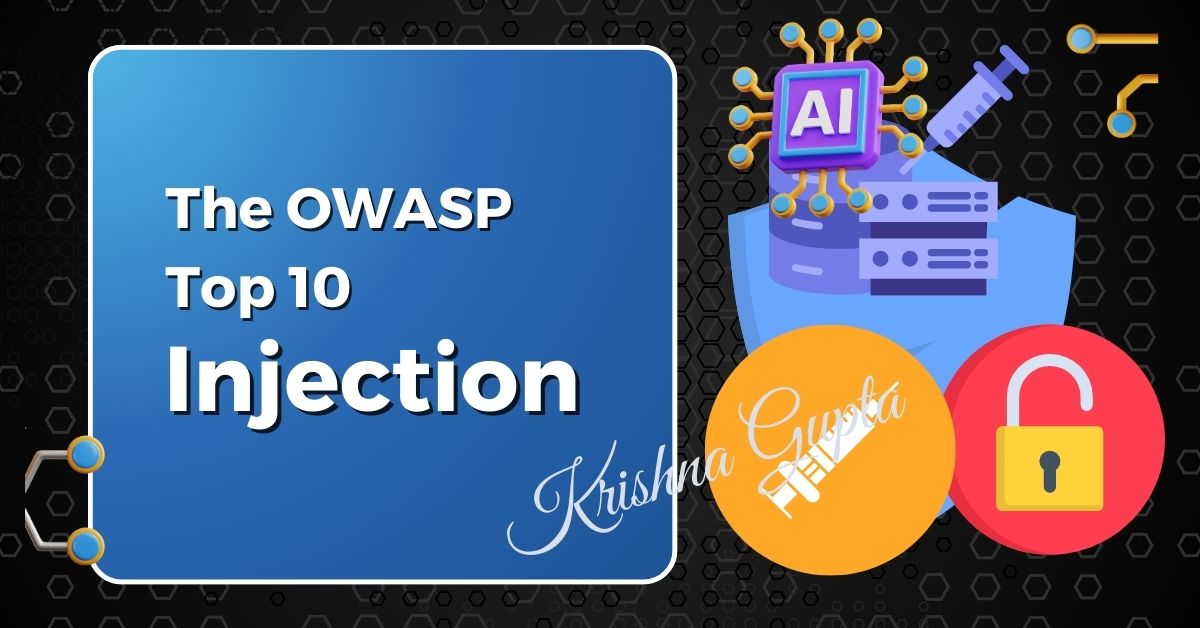
Final Thoughts
Preventing injection vulnerabilities requires a multi-faceted approach that spans secure coding, structured development processes, and rigorous testing. Secure code reviews identify flaws early, an SDLC approach ensures security is integral throughout development, and penetration testing validates the application’s robustness against real-world attacks. By adopting these practices, software developers can build applications that are not only functional but also resilient against injection attacks, safeguarding users and organisational data.