2024 CWE Top 25 Most Dangerous Software Weaknesses: Use After Free CWE-416
Introduction
In 2024, the Common Weakness Enumeration (CWE) organisation released its list of the Top 25 Most Dangerous Software Weaknesses, highlighting the vulnerabilities that pose the greatest risks to systems, businesses, and end-users worldwide. Among these is CWE-416: Use After Free (UAF), a particularly insidious software vulnerability that continues to challenge software developers and penetration testers alike. This blog post delves into the intricacies of CWE-416, offering an in-depth exploration of its causes, impact, detection, and mitigation.
This article aims to provide practical insights, real-world examples, and actionable strategies for software developers and penetration testers to strengthen their defences against UAF vulnerabilities.
Understanding Use After Free (CWE-416)
What Is Use After Free?
At its core, a Use After Free vulnerability occurs when a program continues to use memory after it has been freed or deallocated. This behaviour can result in undefined behaviour, ranging from crashes and data corruption to critical security breaches, including arbitrary code execution.
In simpler terms, imagine a rental property that has been vacated but is still being used by the previous tenant. In the context of programming, this “tenant” could be malicious code exploiting the freed memory space to wreak havoc.
Causes of Use After Free Vulnerabilities
- Programming Errors in Memory Management
- Manual memory management in languages such as C and C++ can lead to errors where developers inadvertently access or modify memory locations that have been freed.
- Dangling Pointers
- Pointers referencing memory locations that have already been freed remain a common culprit. Operations performed on these dangling pointers trigger undefined behaviour.
- Concurrency Issues
- Multi-threaded applications can amplify UAF vulnerabilities when one thread frees memory while another simultaneously accesses it.
- Logic Errors in Object Lifecycles
- Failure to manage the lifecycle of objects, especially in complex systems, can leave memory in a state where it is reused improperly.
Real-World Impacts of Use After Free
The consequences of CWE-416 vulnerabilities extend beyond mere technical disruptions. They have profound implications for businesses, governments, and individuals:
- High-Profile Attacks: In 2021, Google Chrome faced a zero-day exploit linked to a UAF vulnerability in its JavaScript engine. This incident highlighted the real-world consequences of UAF weaknesses, as attackers leveraged the flaw to gain control of affected systems.
- Financial and Reputational Damage: Organisations suffering from UAF exploits may face regulatory fines, legal action, and irreparable brand damage.
- Increased Attack Surface for Malicious Actors: A UAF vulnerability can serve as a stepping stone for advanced persistent threats (APTs), allowing attackers to execute arbitrary code, bypass security controls, or launch further attacks.
Detection and Exploitation: Insights for Penetration Testers
How Attackers Exploit Use After Free Vulnerabilities
- Crafting Malicious Payloads
- Exploit developers often craft payloads to replace the freed memory with malicious data, enabling arbitrary code execution.
- Heap Spraying
- By filling the memory heap with controlled data, attackers increase the likelihood that their payload will occupy the freed space.
- Exploiting Undefined Behaviour
- Undefined behaviour caused by UAF can result in predictable patterns, which skilled attackers exploit for precise targeting.
Tools for Identifying UAF Vulnerabilities
Penetration testers can leverage several tools to uncover UAF issues:
- AddressSanitizer (ASan): A fast memory error detector used to identify memory corruption bugs, including UAF vulnerabilities.
- Valgrind: An instrumentation framework for detecting memory mismanagement.
- Static Analysis Tools: Tools like Coverity and SonarQube analyse source code for potential UAF issues.
- Fuzz Testing: By injecting malformed or unexpected inputs, fuzzers can trigger UAF conditions.
Mitigation Strategies for Developers
- Adopt Memory-Safe Programming Practices
- Languages like Rust or Java that abstract memory management significantly reduce the risk of UAF vulnerabilities.
- Leverage Smart Pointers
- In C++ development, smart pointers like
std::shared_ptr
andstd::unique_ptr
ensure memory is automatically deallocated when no longer needed.
- In C++ development, smart pointers like
- Implement Clear Ownership Rules
- Establishing clear rules about which function or thread owns a resource can prevent premature deallocation.
- Use Modern Compilers and Libraries
- Many modern compilers include security checks that can help identify and prevent UAF vulnerabilities during development.
- Regular Code Reviews and Audits
- Conduct thorough code reviews focusing on memory allocation and deallocation practices.
- Enable Runtime Defences
- Techniques such as Control Flow Integrity (CFI) and Data Execution Prevention (DEP) can mitigate the exploitation of UAF vulnerabilities.
Business Impact and ROI of Addressing UAF
For C-level executives, the cost of mitigating UAF vulnerabilities must be weighed against the potential losses from an exploit:
- Proactive Risk Management: Investing in secure development practices and penetration testing reduces the likelihood of a breach, preserving customer trust and avoiding regulatory fines.
- Operational Continuity: UAF-related exploits can disrupt business operations. Preventative measures ensure uninterrupted service delivery.
- Enhanced Market Competitiveness: Organisations prioritising software security often gain a competitive edge by demonstrating commitment to robust security protocols.
Case Study: Mitigating UAF Vulnerabilities in a Large-Scale Application
Consider a hypothetical case involving a multinational financial services firm that identified a UAF vulnerability in its trading platform. The vulnerability was discovered during routine penetration testing:
- Discovery:
- A penetration tester used AddressSanitizer to identify a dangling pointer in the platform’s transaction processing module.
- Impact Assessment:
- Exploiting the vulnerability could allow attackers to alter transaction records, potentially leading to financial fraud.
- Resolution:
- Developers refactored the affected code to implement smart pointers and eliminate manual memory management.
- Outcome:
- The vulnerability was patched within two weeks, and the company enhanced its software development lifecycle with stricter memory safety practices.
Future Trends in Addressing Use After Free Vulnerabilities
As software systems grow in complexity, addressing UAF vulnerabilities requires a multi-faceted approach:
- AI-Powered Code Analysis:
- Machine learning models trained on vast datasets can identify UAF patterns more effectively than traditional tools.
- Memory-Safe Languages:
- The adoption of Rust and similar languages in critical systems is expected to grow, reducing the prevalence of UAF issues.
- Enhanced Awareness and Training:
- Continuous training for developers and testers will be critical in maintaining awareness of evolving threats.
By equipping developers and testers with the knowledge and tools to combat UAF vulnerabilities, we move closer to a safer, more secure software ecosystem.
Examples of Vulnerable and Secure Memory Management Practices in C++
Example 1: Vulnerable Memory Management
This example demonstrates a Use After Free (UAF) vulnerability caused by improper memory management.
#include <iostream>
void vulnerableFunction() {
int* ptr = new int(42); // Allocate memory on the heap
std::cout << "Value: " << *ptr << std::endl;
delete ptr; // Free the allocated memory
std::cout << "Memory freed." << std::endl;
// Attempt to access the freed memory
std::cout << "Accessing freed memory: " << *ptr << std::endl; // Undefined behaviour
}
int main() {
vulnerableFunction();
return 0;
}
Explanation of Vulnerability:
- Memory is allocated dynamically using
new
. - The memory is freed using
delete
. - The code attempts to dereference the dangling pointer (
ptr
), leading to undefined behaviour, which could potentially be exploited by an attacker.
Example 2: Secure Memory Management Using Smart Pointers
This example demonstrates a safe approach using std::unique_ptr
, which automatically manages the memory lifecycle.
#include <iostream>
#include <memory> // For smart pointers
void secureFunction() {
// Use a smart pointer for automatic memory management
std::unique_ptr<int> ptr = std::make_unique<int>(42);
std::cout << "Value: " << *ptr << std::endl;
// Memory is automatically freed when ptr goes out of scope
}
int main() {
secureFunction();
return 0;
}
Advantages of Smart Pointers:
- Automatic Memory Management: The
std::unique_ptr
automatically deletes the memory when it goes out of scope, preventing manual errors. - No Dangling Pointers: Smart pointers ensure that freed memory cannot be accessed again, thus eliminating UAF vulnerabilities.
Example 3: Secure Memory Management with Explicit Nullifying
If smart pointers cannot be used, explicitly nullifying pointers after freeing memory is a safe alternative.
#include <iostream>
void secureFunction() {
int* ptr = new int(42); // Allocate memory on the heap
std::cout << "Value: " << *ptr << std::endl;
delete ptr; // Free the allocated memory
ptr = nullptr; // Nullify the pointer
// Attempting to dereference a null pointer results in a clear error
if (ptr) {
std::cout << "Accessing memory: " << *ptr << std::endl;
} else {
std::cout << "Pointer is null; cannot access memory." << std::endl;
}
}
int main() {
secureFunction();
return 0;
}
Best Practices:
- Nullify Pointers: After freeing memory, set the pointer to
nullptr
to prevent accidental use. - Check for Null: Before accessing a pointer, ensure it is not
nullptr
.
Key Takeaways
- Avoid Manual Memory Management: Whenever possible, use smart pointers provided by modern C++.
- Automate Memory Lifecycles: Tools like
std::shared_ptr
andstd::unique_ptr
abstract away the complexities of memory management. - Nullify Dangling Pointers: If manual deallocation is required, nullify pointers immediately after freeing memory to mitigate UAF vulnerabilities.
Simulated Use After Free (UAF) Exploit in C++
The following code demonstrates a simulated Use After Free (UAF) vulnerability. It shows how attackers can manipulate freed memory to inject malicious data, leading to arbitrary code execution or other security breaches. This example is for educational purposes only, emphasising the importance of recognising and mitigating such vulnerabilities.
Simulated Vulnerability
#include <iostream>
class VictimClass {
public:
void displayMessage() {
std::cout << "Safe: This is a valid message." << std::endl;
}
};
void simulatedUAFExploit() {
VictimClass* victim = new VictimClass(); // Allocate memory for an object
victim->displayMessage();
delete victim; // Free the allocated memory
std::cout << "Memory freed." << std::endl;
// Simulate an attacker reusing the freed memory
int* attackerControl = reinterpret_cast<int*>(victim);
*attackerControl = 0xdeadbeef; // Overwrite freed memory with malicious data
// Attempt to call the original object's method (UAF exploit)
victim->displayMessage(); // This triggers undefined behaviour
}
int main() {
simulatedUAFExploit();
return 0;
}
Explanation of the Exploit
- Memory Allocation: A
VictimClass
object is created on the heap. - Memory Deallocation: The
delete
operation frees the memory associated with thevictim
pointer. - Attacker Reuse: The memory space previously occupied by
victim
is overwritten with attacker-controlled data (0xdeadbeef
). - Undefined Behaviour: When the
displayMessage()
method is called on the freed memory, it causes undefined behaviour. This could potentially execute malicious code or crash the program.
Impact of UAF Exploits
- Arbitrary Code Execution: Attackers can inject and execute malicious payloads in the memory space of the freed object.
- System Compromise: UAF vulnerabilities are often used as entry points for advanced threats, such as privilege escalation or system takeover.
- Data Corruption: Critical data structures can be overwritten, leading to application instability or incorrect behaviour.
Mitigating UAF Vulnerabilities
1. Use Smart Pointers Smart pointers like std::unique_ptr
or std::shared_ptr
automatically manage the lifecycle of dynamically allocated memory, preventing UAF scenarios.
#include <iostream>
#include <memory>
void secureExample() {
auto victim = std::make_unique<VictimClass>();
victim->displayMessage();
// Memory is safely managed and cannot be reused by an attacker
}
2. Implement Runtime Defences Enable runtime security mechanisms like AddressSanitizer to detect and halt UAF vulnerabilities during development.
3. Clear and Nullify Pointers After freeing memory, set the pointer to nullptr
to prevent accidental reuse.
Importance of Mitigation
- Business Continuity: Preventing UAF exploits ensures system stability and uninterrupted operations.
- Customer Trust: Safeguarding against vulnerabilities demonstrates a commitment to security, bolstering customer confidence.
- Cost Avoidance: Mitigating UAF vulnerabilities early reduces the likelihood of expensive breaches, downtime, and reputational damage.
By understanding and addressing UAF vulnerabilities, software developers and penetration testers can proactively strengthen system defences, safeguarding against this dangerous weakness.
Detecting Use After Free (UAF) vulnerabilities requires a combination of static and dynamic analysis tools that can identify unsafe memory management practices or runtime errors. Below is a list of tools commonly used by developers and security professionals to detect UAF vulnerabilities.
1. AddressSanitizer (ASan)
- Type: Runtime memory error detector.
- Use Case: ASan is integrated into modern C/C++ compilers like GCC and Clang. It helps detect memory issues, including UAF, buffer overflows, and double frees, at runtime.
- How It Works: ASan instruments the binary to monitor memory access and identify invalid operations.
- Example Usage:
g++ -fsanitize=address -g -o program program.cpp ./program
2. Valgrind
- Type: Dynamic analysis tool.
- Use Case: Detects memory management issues such as UAF, memory leaks, and invalid memory access.
- How It Works: Valgrind runs programs in a virtual machine-like environment, instrumenting each memory operation to catch invalid accesses.
- Example Usage:
valgrind --tool=memcheck ./program
3. Clang Static Analyzer
- Type: Static analysis tool.
- Use Case: Analyses source code to identify potential UAF vulnerabilities and other issues without executing the code.
- How It Works: The tool examines control flow and memory usage patterns to detect bugs.
- Example Usage:
scan-build clang++ program.cpp
4. Coverity Static Analysis
- Type: Commercial static analysis tool.
- Use Case: Detects a wide range of software vulnerabilities, including UAF, during the development process.
- Key Features:
- Supports integration with CI/CD pipelines.
- Provides detailed reports for each vulnerability.
- Website: Coverity
5. Dr. Memory
- Type: Dynamic analysis tool.
- Use Case: Identifies memory-related vulnerabilities such as UAF, memory leaks, and uninitialised reads.
- How It Works: Instruments the binary to detect invalid memory operations.
- Example Usage:
drmemory -- program
6. GDB with Custom Scripts
- Type: Debugger with scripting.
- Use Case: While not specialised, GDB can be scripted to monitor memory allocation and detect UAF conditions during debugging.
- Example Usage:
gdb ./program
7. Helgrind (Valgrind Tool)
- Type: Concurrency analyser.
- Use Case: Detects threading issues that could lead to UAF vulnerabilities in multi-threaded applications.
- How It Works: Instruments memory access patterns across threads.
- Example Usage:
valgrind --tool=helgrind ./program
8. Sanitizers in Modern Compilers
Modern compilers (GCC and Clang) offer a suite of sanitizers that can detect UAF and other vulnerabilities during testing:
- MemorySanitizer: Detects uninitialised memory reads.
- UndefinedBehaviourSanitizer (UBSan): Identifies undefined behaviours like accessing invalid pointers.
Example:
g++ -fsanitize=undefined,address -g -o program program.cpp
./program
9. AFL (American Fuzzy Lop)
- Type: Fuzzer.
- Use Case: Generates malformed inputs to stress-test applications and expose UAF vulnerabilities by triggering crashes.
- How It Works: AFL mutates input files and monitors program behaviour for anomalies.
Example Usage:
afl-fuzz -i input_dir -o output_dir ./program
10. Static Code Analysis IDE Plugins
Popular IDEs such as Visual Studio, JetBrains CLion, and Eclipse offer plugins or built-in tools for static analysis that flag UAF vulnerabilities during development.
Comparison of Tools
Tool | Type | Strengths | Limitations |
---|---|---|---|
AddressSanitizer | Dynamic | Fast, integrated with compilers | Only detects runtime issues |
Valgrind | Dynamic | Detailed memory issue reports | Slower execution |
Clang Static Analyzer | Static | No runtime needed, early detection | May produce false positives |
Coverity | Static | Comprehensive analysis, CI/CD friendly | Commercial tool |
Dr. Memory | Dynamic | Easy to use, good for basic UAF checks | Limited advanced features |
AFL | Fuzzer | Stress-tests programs with malformed inputs | Requires manual crash analysis |
Recommendations
For the best results:
- Use AddressSanitizer or Valgrind during development to catch UAF issues dynamically.
- Integrate Clang Static Analyzer or Coverity into your build pipeline for early detection.
- Perform fuzz testing with tools like AFL to uncover edge-case vulnerabilities.
Difference Between Use After Free (UAF) and Buffer Overflow
While Use After Free (CWE-416) and Buffer Overflow (CWE-120) are both memory-related vulnerabilities, they differ significantly in their causes, mechanisms, and impacts. Below is a detailed comparison:
1. Definition
Aspect | Use After Free (UAF) | Buffer Overflow |
---|---|---|
What It Is | Occurs when a program accesses memory after it has been freed or deallocated. | Occurs when a program writes data beyond the boundaries of a memory buffer, overwriting adjacent memory. |
2. Root Cause
Aspect | Use After Free (UAF) | Buffer Overflow |
---|---|---|
Cause | – Manual memory management errors (e.g., using delete in C++).- Dangling pointers.- Concurrency issues where one thread frees memory that another thread still uses. | – Failure to validate input size.- Writing to a memory buffer without bounds checking.- Mismanagement of array indices or loops. |
3. Trigger Mechanism
Aspect | Use After Free (UAF) | Buffer Overflow |
---|---|---|
Trigger | – Accessing memory that has already been freed.- Invoking methods on objects that no longer exist in memory. | – Writing more data into a buffer than its allocated size.- Reading/writing beyond array or string bounds. |
4. Behaviour
Aspect | Use After Free (UAF) | Buffer Overflow |
---|---|---|
Behaviour | – Causes undefined behaviour.- May allow attackers to inject malicious data into the freed memory space.- Can lead to crashes, data corruption, or code execution. | – Overwrites adjacent memory, leading to crashes, corruption of data structures, or control flow hijacking.- Often used to inject and execute malicious code. |
5. Exploitation
Aspect | Use After Free (UAF) | Buffer Overflow |
---|---|---|
Exploitation | – Attackers can “reuse” the freed memory and replace its content with malicious data.- Exploits often involve heap spraying or precise memory manipulation. | – Attackers overwrite function return addresses, pointers, or adjacent buffers to hijack control flow.- Exploits are often stack-based or heap-based. |
6. Common Scenarios
Aspect | Use After Free (UAF) | Buffer Overflow |
---|---|---|
Examples | – A dangling pointer accesses freed memory in C++. Example: |
int* ptr = new int(42); delete ptr; *ptr = 5; // UAF``` | - Writing past the end of an array in C.
Example:
```cpp
char buffer[10]; strcpy(buffer, "This string is too long"); // Overflow``` |
---
### **7. Detection**
| **Aspect** | **Use After Free (UAF)** | **Buffer Overflow** |
|-----------------------|---------------------------------------------------|--------------------------------------------|
| **Detection Tools** | - AddressSanitizer (ASan).<br>- Valgrind.<br>- Static analysis tools (e.g., Coverity). | - AddressSanitizer (ASan).<br>- Fuzzing tools (e.g., AFL).<br>- Boundary checkers (e.g., StackGuard). |
---
### **8. Mitigation**
| **Aspect** | **Use After Free (UAF)** | **Buffer Overflow** |
|-----------------------|---------------------------------------------------|--------------------------------------------|
| **Mitigation** | - Use smart pointers (`std::unique_ptr`, `std::shared_ptr`).<br>- Always nullify freed pointers.<br>- Automate memory management with languages like Rust. | - Validate input lengths rigorously.<br>- Use functions that limit buffer writes (e.g., `strncpy` instead of `strcpy`).<br>- Implement runtime protections like Stack Canaries and Data Execution Prevention (DEP). |
---
### **9. Business Impact**
| **Aspect** | **Use After Free (UAF)** | **Buffer Overflow** |
|-----------------------|---------------------------------------------------|--------------------------------------------|
| **Business Impact** | - Exploits often target critical applications like browsers, enabling data theft or system compromise.<br>- UAF vulnerabilities are frequently exploited in high-profile attacks, e.g., Chrome vulnerabilities. | - Buffer overflows are one of the most exploited vulnerabilities in cybersecurity history.<br>- Attackers can gain unauthorised access, steal sensitive data, or crash critical systems. |
---
### **10. Example Scenarios**
#### **Use After Free**
- A web browser allocates memory for a DOM element but fails to clean up dangling references after freeing it, leading to UAF when the element is accessed again.
#### **Buffer Overflow**
- A C-based application accepts unchecked user input, writing beyond a buffer's limit, overwriting the return address of a function, and allowing an attacker to execute malicious shellcode.
---
### **Summary**
| **Feature** | **Use After Free (UAF)** | **Buffer Overflow** |
|-----------------------|---------------------------------------------------|--------------------------------------------|
| **Focus** | Improper use of freed memory. | Writing outside allocated memory bounds. |
| **Complexity** | Requires precise memory manipulation by the attacker. | Often easier to exploit but highly impactful. |
Both vulnerabilities represent serious risks to software security. While their causes and mechanisms differ, the solution lies in rigorous programming practices, memory-safe languages, and robust testing methodologies.
Final Thoughts
Use After Free (CWE-416) remains one of the most dangerous software vulnerabilities in 2024, posing risks to systems, organisations, and end-users alike. By understanding the underlying causes, recognising the tactics used by attackers, and implementing robust defences, software developers and penetration testers can significantly mitigate these risks.
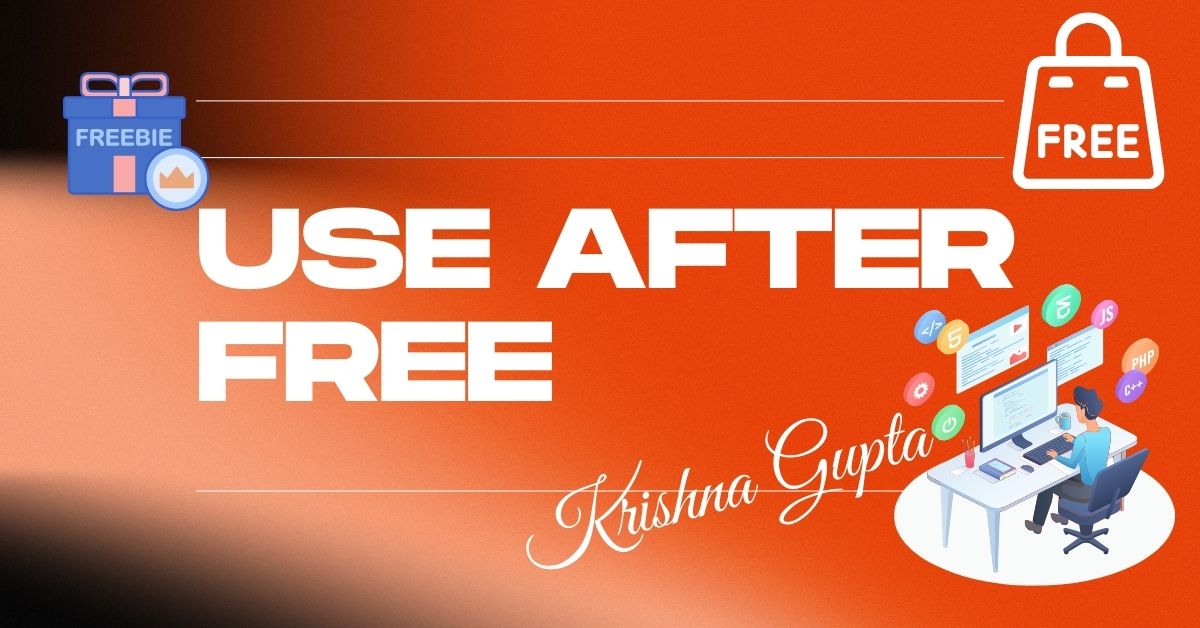
For businesses, addressing UAF vulnerabilities is not just a technical imperative but a strategic necessity. The stakes are high, but with the right tools, training, and commitment to secure practices, it is possible to stay ahead of adversaries and safeguard the integrity of critical systems.